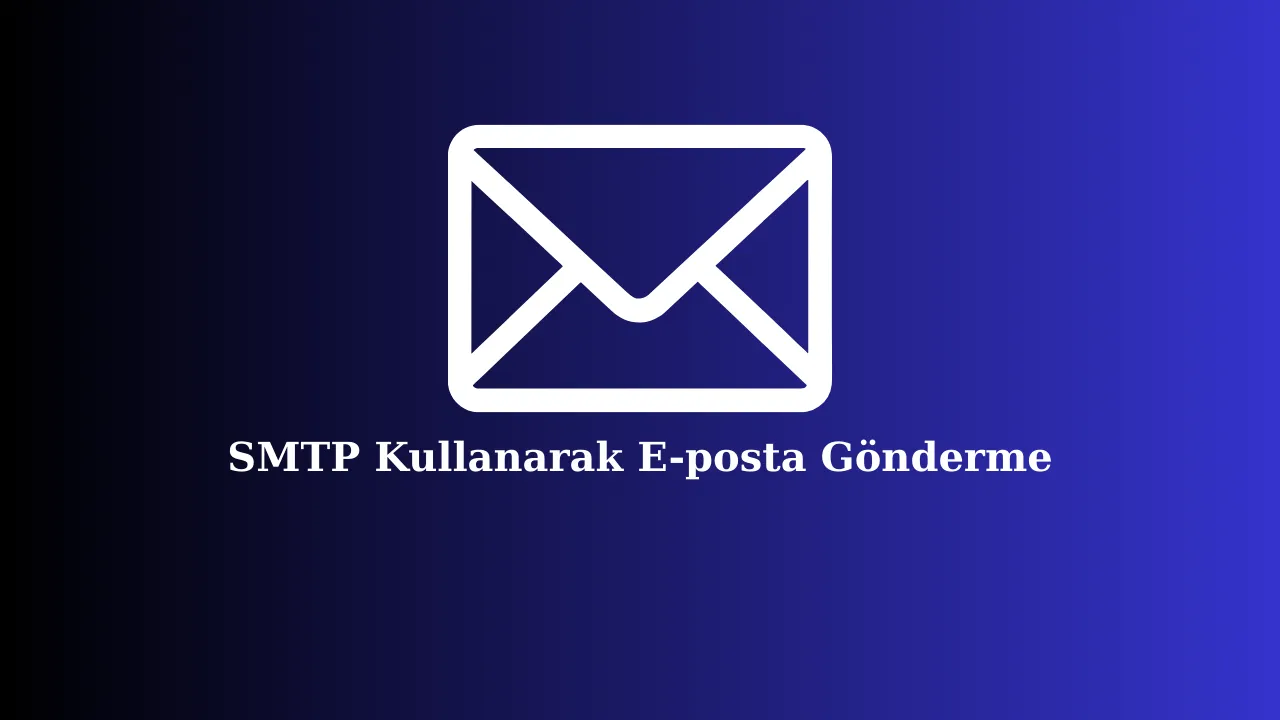
Sending Email Using SMTP
İçindekiler
Sending Email Using SMTP
Sending emails programmatically is a common requirement for many applications. Whether it's for user registration, password resets, notifications, or marketing campaigns, the ability to reliably send emails is crucial. One of the most common and reliable ways to send email is through the Simple Mail Transfer Protocol (SMTP).
What is SMTP?
SMTP (Simple Mail Transfer Protocol) is a standard protocol for sending emails across the internet. It's the protocol that email clients (like Outlook, Gmail, Thunderbird) use to send messages to an email server, which then relays the message to the recipient's email server. Understanding SMTP is key to programmatically sending emails successfully.
Why Use SMTP?
While there are other ways to send emails (like using a web API), SMTP offers several advantages:
- Reliability: SMTP is a well-established and reliable protocol.
- Flexibility: You can configure SMTP servers to meet your specific needs.
- Control: You have more control over the sending process, including authentication and encryption.
- Deliverability: Properly configured SMTP servers can improve email deliverability, reducing the chances of your emails ending up in spam folders.
How SMTP Works
The basic SMTP process involves the following steps:
- Connection: Your application connects to an SMTP server on a specific port (typically 25, 465, or 587).
- Authentication: You authenticate with the SMTP server using a username and password (if required).
- Transaction: You initiate a mail transaction, specifying the sender, recipient, subject, and body of the email.
- Data Transfer: You transfer the email data to the SMTP server.
- Termination: The SMTP server processes the email and relays it to the recipient's email server.
Setting Up an SMTP Server
You have several options for setting up an SMTP server:
- Use a Third-Party SMTP Service: Services like SendGrid, Mailgun, Amazon SES, and Postmark provide reliable SMTP servers and email delivery management tools. This is often the easiest and most reliable option, especially for production environments.
- Configure Your Own SMTP Server: You can set up your own SMTP server using software like Postfix, Exim, or Microsoft Exchange. This requires more technical expertise but gives you complete control over your email infrastructure.
Sending Email with Code (Python Example)
Here's a simple example of how to send an email using Python's smtplib
library:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# Email configuration
sender_email = "[email protected]"
sender_password = "your_password" # Use App Password if using Gmail
receiver_email = "[email protected]"
smtp_server = "smtp.example.com"
smtp_port = 587 # or 465 for SSL
# Create the email message
message = MIMEMultipart("alternative")
message["Subject"] = "Hello from Python!"
message["From"] = sender_email
message["To"] = receiver_email
# Create the plain-text and HTML versions of the message
text = """\
Hi,
How are you?
This is a test email sent using SMTP.
"""
html = """\
Hi,
How are you?
This is a test email sent using SMTP.
"""
# Turn these into plain/html MIMEText objects
part1 = MIMEText(text, "plain")
part2 = MIMEText(html, "html")
# Add HTML/plain-text parts to MIMEMultipart message
# The email client will try to render the last part first
message.attach(part1)
message.attach(part2)
# Send the email
try:
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # Secure the connection
server.login(sender_email, sender_password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully!")
except Exception as e:
print(f"Error sending email: {e}")
finally:
if 'server' in locals():
server.quit()
Important Notes:
- Security: Always use a secure connection (TLS/SSL) when sending emails.
- Gmail App Passwords: If you are using Gmail, you may need to enable "less secure app access" or create an "App Password" in your Google account settings. App Passwords are highly recommended for security.
- Error Handling: Implement proper error handling to catch and log any exceptions that occur during the sending process.
- Rate Limiting: Be mindful of rate limits imposed by your SMTP server to avoid being blocked.
- Email Formatting: Use the
email.mime
modules to properly format your emails, including headers, attachments, and HTML content.
Troubleshooting Common SMTP Issues
Here are some common issues you might encounter when sending emails with SMTP:
- Connection Refused: The SMTP server is not accessible. Check the server address and port.
- Authentication Failed: Incorrect username or password. Double-check your credentials.
- Relay Access Denied: The SMTP server is not configured to allow relaying from your IP address. Contact your SMTP provider.
- Email Marked as Spam: Your email content may be triggering spam filters. Review your email content and ensure you are following best practices for email deliverability. Check your domain's SPF, DKIM, and DMARC records.
Conclusion
Sending emails using SMTP is a fundamental skill for developers. By understanding the principles of SMTP and using appropriate libraries and tools, you can reliably send emails from your applications. Remember to prioritize security, error handling, and email deliverability to ensure your emails reach their intended recipients.
Yorumlar